Mastering TypeScript: Utilizing Conditional Types for Enhanced Type Safety
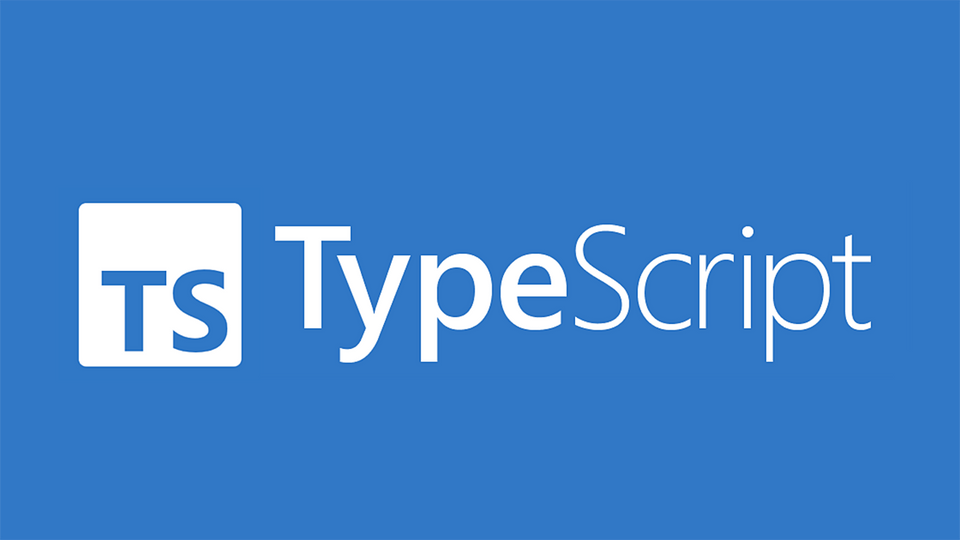
Introduction
TypeScript has gained immense popularity among developers for its ability to bring static typing to JavaScript, enabling better code quality and improved developer experience. With its powerful type system, TypeScript offers several advanced features that can take your coding skills to the next level. In this blog post, we'll explore one such feature: conditional types. We'll dive into how conditional types work and demonstrate their practical applications in enhancing type safety.
Understanding Conditional Types:
Conditional types are a fundamental part of TypeScript's type system and allow us to perform type inference and transformation based on a condition. These types enable us to define types that depend on other types, making them highly versatile in complex scenarios.
Syntax
The syntax for conditional types follows the form T extends U ? X : Y, where T, U, X, and Y are type expressions. It can be read as "if T is assignable to U, then the type is X; otherwise, it is Y."
Practical Use Cases
Discriminating Union Types:
Conditional types are particularly useful when working with discriminated union types. Consider a scenario where you have an Animal interface with subtypes Cat and Dog. You can utilize a conditional type to extract a specific subtype based on a discriminator property.
typescript
interface Animal {
type: 'cat' | 'dog';
// ...
}
type GetAnimalSound<T extends Animal> = T['type'] extends 'cat'
? 'Meow'
: 'Woof';
const catSound: GetAnimalSound<Cat> = 'Meow'; // Type checks
const dogSound: GetAnimalSound<Dog> = 'Woof'; // Type checks
Infer Function Return Types:
You can leverage conditional types to infer the return type of a function dynamically. This is particularly useful when dealing with libraries or frameworks that use higher-order functions extensively.
typescript
Copy code
function getResult<T>(value: T): T extends Promise<infer R> ? R : T {
if (value instanceof Promise) {
return value.then((result) => result) as T extends Promise<infer R> ? R : T;
}
return value;
}
const promiseResult = getResult(fetchData()); // Infers the promise result type
const normalResult = getResult(42); // Infers the type as number
Type Mapping and Transformation
Conditional types can be used for type mapping and transformation based on a condition. Let's say you have an array of values that may or may not be promises. You can use a conditional type to convert all promise values into their resolved types, while keeping the non-promise values intact.
typescript
type ResolvePromises<T> = T extends Promise<infer R> ? R : T;
function resolveArray<T>(array: T[]): ResolvePromises<T>[] {
return array.map((item) =>
item instanceof Promise ? item.then((result) => result) : item
) as ResolvePromises<T>[];
}
const values = [Promise.resolve('Hello'), 'World', Promise.resolve(42)];
const resolvedValues = resolveArray(values); // Resolves promises and keeps non-promise values
console.log(resolvedValues); // Output: ['Hello', 'World', 42]
Conclusion
Conditional types offer an incredibly powerful toolset for enhancing type safety and enabling advanced type inference in TypeScript. By harnessing their capabilities, you can write more robust code, prevent runtime errors, and unlock a whole new level of flexibility within the TypeScript ecosystem. Whether you're dealing with discriminated union types, dynamically inferring function return types, or performing type mapping and transformation, conditional types are a valuable addition to your TypeScript toolkit.
Remember to experiment, explore the TypeScript documentation, and practice implementing conditional types in your projects. Embrace the possibilities they bring, and you'll find yourself writing safer and more reliable TypeScript code. Happy typing!